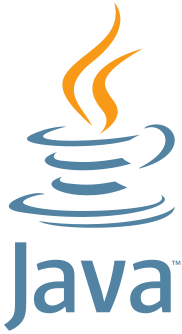
Introduction
Landing a role as a Java Developer is an exciting step in your career, opening doors to a wide range of development opportunities. With Java being a foundational language for enterprise applications, employers are on the lookout for skilled developers who understand core Java concepts, object-oriented programming (OOP) principles, and best practices for building scalable applications. This guide covers essential topics, questions, and expert tips to help you prepare for your Java Developer interview with confidence.
Essential Java Knowledge Areas
Understand Java’s Core Concepts and FeaturesStart by mastering Java’s key features, including platform independence (JVM, JRE, JDK), object-oriented programming principles (encapsulation, inheritance, polymorphism, and abstraction), and the significance of Java’s memory management with garbage collection. Explain how Java’s “write once, run anywhere” philosophy and its robust security model make it a popular choice.
Object-Oriented Programming (OOP) PrinciplesDive deep into the four pillars of OOP in Java—encapsulation, inheritance, polymorphism, and abstraction. Be prepared to discuss how each principle is applied in Java applications, with examples illustrating classes, interfaces, inheritance hierarchies, and polymorphic behavior using method overloading and overriding.
Java Collections FrameworkJava’s Collections Framework is vital for data handling. Familiarize yourself with key interfaces like List, Set, and Map, and their implementations (e.g., ArrayList, LinkedList, HashSet, HashMap). Explain how to choose the right collection based on performance needs, and discuss operations such as sorting, filtering, and iterating through collections.
Exception Handling in JavaDiscuss Java’s structured approach to handling runtime errors using try-catch-finally blocks, throw and throws keywords, and custom exceptions. Be prepared to explain the difference between checked and unchecked exceptions, and how to use best practices for exception handling to ensure clean and robust code.
Java Multithreading and ConcurrencyMultithreading is crucial for efficient Java applications. Explain how Java supports multithreading using the Thread class and Runnable interface. Be familiar with concurrency utilities in java.util.concurrent, such as ExecutorService, Callable, Future, and Synchronization techniques (e.g., synchronized keyword, locks) to manage concurrent tasks safely and efficiently.
Advanced Topics for Java Developers
Java Streams and Lambda Expressions (Java 8+)Java 8 introduced functional programming features, including Streams and lambda expressions. Explain how Streams allow for processing collections in a functional style, enabling operations like filtering, mapping, and reducing data. Discuss how lambda expressions enhance code readability and flexibility by allowing concise function definitions.
Java Memory Management and Garbage CollectionUnderstanding Java’s memory model is key. Describe the heap and stack memory structure, the lifecycle of objects, and how Java’s garbage collector (GC) works. Discuss different GC algorithms (e.g., Serial, Parallel, CMS, G1) and tuning techniques for optimal memory management and application performance.
Spring Framework and Dependency InjectionThe Spring Framework is widely used in Java development. Explain core concepts like Inversion of Control (IoC), dependency injection (DI), and Spring modules (e.g., Spring MVC, Spring Boot). Familiarize yourself with configuring beans, understanding the application context, and creating REST APIs with Spring Boot to demonstrate your knowledge of building scalable applications.
Database Interaction with JDBC and ORM ToolsMost Java applications interact with databases. Describe how Java connects to databases using JDBC, how to perform CRUD operations, and the importance of prepared statements for security. Familiarize yourself with ORM tools like Hibernate, JPA (Java Persistence API), and how they simplify database interactions in Java applications.
RESTful API Design and ImplementationREST APIs are fundamental for web and mobile apps. Be prepared to discuss REST principles, HTTP methods (GET, POST, PUT, DELETE), and how to create RESTful services using Spring Boot. Mention how to handle JSON data, HTTP status codes, and best practices for designing secure and efficient APIs.
Behavioral and Scenario-Based Questions
Describe a Time You Optimized Java Application PerformanceShare an example of a project where you identified and resolved performance bottlenecks. Mention specific tools or techniques, such as profiling, garbage collection tuning, or optimizing SQL queries. Highlight any tangible improvements in response time or resource usage.
Explain a Challenging Bug You Encountered and SolvedDescribe a complex bug, such as a concurrency issue or a memory leak. Outline your troubleshooting steps, the tools you used (e.g., debugger, logs), and how you resolved it. Mention any preventative measures you implemented to avoid similar issues in the future.
How Do You Approach Writing Clean, Maintainable Code?Discuss your coding standards, such as following the SOLID principles, using design patterns, and avoiding anti-patterns. Mention practices like code reviews, automated testing, and continuous refactoring to ensure code readability and maintainability.
Share Your Experience with Version Control (e.g., Git)Version control is crucial for collaborative development. Explain how you use Git for branching, merging, and resolving conflicts. Mention best practices for commit messages and workflows (e.g., Git Flow), showing your experience in working in a team environment.
How Do You Stay Updated with Java Technologies?Highlight your commitment to continuous learning by mentioning resources like Oracle documentation, Java forums (Stack Overflow, JavaWorld), online courses, and certifications like Oracle Certified Professional (OCP) in Java. Staying current with these resources shows your dedication to keeping up with Java’s evolution.
Additional Preparation Tips
Hands-On Practice: Set up a local development environment or use platforms like LeetCode and HackerRank to practice Java coding, data structures, algorithms, and typical programming problems.
Gain Familiarity with Cloud Platforms: As cloud computing becomes prevalent, knowing how to deploy Java applications on cloud services like AWS, Google Cloud Platform (GCP), and Azure can be beneficial.
Pursue Java Certifications: Earning certifications like Oracle Certified Associate (OCA) and Oracle Certified Professional (OCP) in Java can demonstrate your expertise and commitment to the Java platform.
Conclusion
Preparing for a Java Developer interview requires a blend of technical expertise, problem-solving skills, and understanding of real-world application scenarios. By mastering core Java concepts, exploring advanced frameworks, and practicing coding problems, you can confidently approach your interview. Review these questions, stay updated on Java trends, and you’ll be ready to impress in your Java Developer interview and advance in your software development career.
Comments